Then users can request permission to the site using RequestAccess page. In SharePoint 2010, master pages are not applied to this application page. What we can do here is we can create a custom RequestAccess page with our own fields. Then we can use Set-SPCustomLayoutsPage Power Shell Command to map a new path for the custom RequestAccess layout page as follows:
Set-SPCustomLayoutsPage –Identity "RequestAccess" –RelativePath "/_layouts/custompages/MyRequestAccess.aspx" –WebApplication "http://server_name/mywebapp"
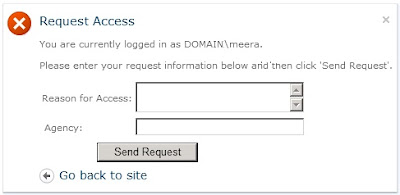
This is the implementation of MyRequestAccess custom page. It is implemented to support access requests for SP sites as well as SP lists.
public partial class MyRequestAccess : UnsecuredLayoutsPageBase
{
private string m_strListID;
protected void Page_Load(object sender, EventArgs e)
{
// Check whether the access request permission is enabled.
if (!base.Web.RequestAccessEnabled)
{
// Redirect to Error Page.
}
if (base.Request.QueryString["type"] == "list")
{
this.m_strListID = base.Request.QueryString["name"];
if (!IsGuid(this.m_strListID))
{
// Redirect to Error Page.
}
else
{
SPSecurity.CodeToRunElevated secureCode = null;
if (secureCode == null)
{
secureCode = delegate
{
using (SPSite site = new SPSite(
new Uri(base.Site.Url).ToString(), SPUserToken.SystemAccount))
{
using (SPWeb web = site.OpenWeb(base.Web.ServerRelativeUrl))
{
SPList list = web.Lists[new Guid(this.m_strListID)];
if (!list.RequestAccessEnabled)
{
// Redirect to Error Page.
}
}
}
};
}
SPSecurity.RunWithElevatedPrivileges(secureCode);
}
}
}
protected void btnSubmit_OnClick(object sender, EventArgs e)
{
// Check access request is for a list or the site.
bool isSite = this.m_strListID == null ? true: false;
try
{
string parentSiteWithUniquePermissionsUrl = SPUtility.GetFullUrl
(base.Web.Site, base.Web.FirstUniqueAncestorWeb.ServerRelativeUrl);
string siteUrl = SPUtility.GetFullUrl(base.Web.Site,
base.Web.ServerRelativeUrl);
// Read user povided values.
string reason = SPHttpUtility.HtmlEncode(txtReason.Text);
string agencyCode = SPHttpUtility.HtmlEncode(txtAgencyCode.Text);
string parentSiteWithUniquePermissionsLayoutsUrl =
String.Concat(parentSiteWithUniquePermissionsUrl, "/_layouts/");
string siteLayoutsUrl = String.Concat(siteUrl, "/_layouts/");
// Get user name.
string userName = string.Empty;
if (base.Site.WebApplication.GetIisSettingsWithFallback(
base.Site.Zone).UseClaimsAuthentication)
{
userName = SPClaimProviderManager.Local.GetUserIdentifierEncodedClaim(
this.Context.User.Identity);
}
else
{
userName = this.Context.User.Identity.Name;
}
string encodedUserName = SPHttpUtility.UrlKeyValueEncode(userName);
// Build access granting link.
string grantAccessLink = null;
if (isSite)
{
grantAccessLink = "<a href = \"" +
SPHttpUtility.UrlPathEncode(parentSiteWithUniquePermissionsLayoutsUrl
+ "aclinv.aspx", true) + "?users=" + encodedUserName + "\">";
}
else
{
grantAccessLink = "<a href = \"" +
SPHttpUtility.UrlPathEncode(siteLayoutsUrl + "aclinv.aspx", true) +
"?users=" + encodedUserName + "&obj=" +
SPHttpUtility.UrlKeyValueEncode(this.m_strListID) + ",list\">";
}
// Create message body "string messageBody" //
// Send Email.
return SPUtility.SendRequestAccessToOwner(base.Web, isSite ?
SPObjectType.Web : SPObjectType.List, this.m_strListID, messageBody);
}
catch (Exception)
{ }
// Redirect to confirmation page.
SPUtility.Redirect("confirmation.aspx?ConfirmationID=RequestAccessSent",
SPRedirectFlags.RelativeToLayoutsPage, this.Context);
}
}
1 comment:
Thanks for wrriting this
Post a Comment